Bitcoin
Get Private Key – Works with the Bitcoin Address Python library by converting compressed WIF to uncompressed WIF.
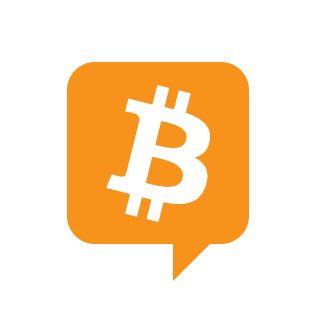
To convert a compressed WIF to an uncompressed WIF, you need to do the following:
- Decode compressed WIF
- Private key extraction
- remove suffix
- Recalculate checksum
- Re-encode to Base58
One way this could be implemented is as follows:
def decompress_WIF(compressed_wif):
hex_data = binascii.b2a_hex(base58.b58decode(compressed_wif)).decode()
if len(hex_data) != 76:
raise ValueError("Invalid length for a compressed WIF")
prefix = hex_data(:2)
suffix = hex_data(-10:-8)
original_checksum = hex_data(-8:)
xpriv = hex_data(2:-10)
if suffix != "01":
raise ValueError("The provided WIF is not a compressed key")
if checksum(prefix + xpriv + suffix) != original_checksum:
raise ValueError("Invalid checksum in the provided WIF")
new_checksum = checksum(prefix + xpriv)
uncompressed_wif = base58.b58encode(binascii.a2b_hex(prefix + xpriv + new_checksum)).decode()
return uncompressed_wif
This code doesn’t check the version byte at all.