Bitcoin
wallet – How to create and sign transactions locally in Ruby
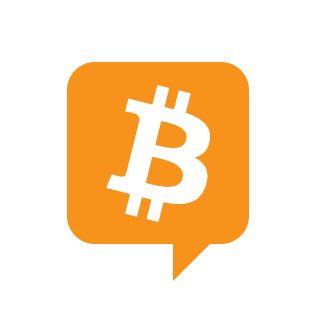
So I’m using public node for Bitcoin node integration and previously I used sendtoaddress method to build, sign and broadcast transactions on the node. But now the sendtoaddress method is not allowed on public nodes due to privacy reasons due to private key disclosure, so we have to create, sign and broadcast the transaction locally offline. I’m using Ruby on Rails (openware/peatio). Here is my code so far: I am using the Bitcoinrb gem ( https://rubygems.org/gems/bitcoinrb ).
TESTNET_BASE_URL = "https://blockstream.info/testnet/api/address/".freeze
MAINNET_BASE_URL = "https://blockstream.info/api/address/".freeze
def create_transaction!(transaction, options = )
env = @currency.dig(:key)&.include?('testnet') ? "testnet" : "mainnet"
::Bitcoin.chain_params=(env)
key = ::Bitcoin::Key.new(priv_key: @wallet.fetch(:secret))
url = @currency.dig(:key)&.include?('testnet') ? TESTNET_BASE_URL : MAINNET_BASE_URL
response = URI.open(url + "#@wallet(:address)/utxo")
utxos = JSON.parse(response.read)
trx = build_trx(utxos.first, transaction.to_address, transaction.amount)
trx = sign_transaction(trx, @wallet.fetch(:secret))
signed_trx = client.json_rpc(:sendrawtransaction, "hexstring": trx.to_payload.bth, maxfeerate: 0)
Rails.logger.warn "-=-=-=sendrawtransaction-=-=- #signed_trx.inspect -=-=-=-=-=-=-=-="
signed_trx
rescue Bitcoind::Client::Error => e
raise Peatio::Wallet::ClientError, e
end
def sign_transaction(tx, private_key)
key = Bitcoin::Key.new(priv_key: private_key)
tx.inputs.each_with_index do |input, index|
script_pubkey = Bitcoin::Script.to_p2pkh(key.pubkey)
input.script_sig = script_pubkey
end
end
def build_trx(utxo, recipient_address, amount)
tx = Bitcoin::Tx.new
tx_in = Bitcoin::TxIn.new(out_point: Bitcoin::OutPoint.new(utxo("txid"), utxo("vout").to_i))
tx.inputs << tx_in
tx_out = Bitcoin::TxOut.new(value: amount.to_f, script_pubkey: Bitcoin::Script.to_p2pkh(recipient_address))
tx.outputs << tx_out
tx
end
However, the output of sendrawtrasnaction is:
"statusCode":403,"errorCode":"btc.blockchain.broadcast.error","message":"Unable to broadcast transaction.","cause":"Request failed with status code 500","dashboardLog":"https://dashboard.tatum.io/logs?id=663f683a798bb0f969fc3760"
Please let me know if I need to do it any other way. If I’m doing something wrong or missing something, I’d appreciate some help.