Bitcoin
Cryptography – How to convert private key to Ethereum address using Python
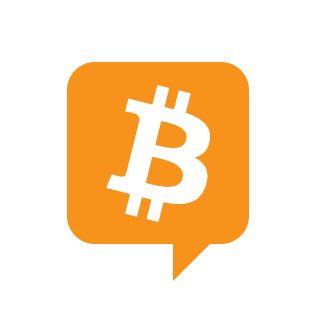
This is my code.
from eth_keys import keys
from eth_utils import decode_hex, encode_hex, keccak
def private_key_to_address(private_key_hex):
# Ensure the private key is a valid hex string
if private_key_hex.startswith('0x'):
private_key_hex = private_key_hex(2:)
# Convert the hex private key to bytes
private_key_bytes = bytes.fromhex(private_key_hex)
# Create a PrivateKey object
private_key = keys.PrivateKey(private_key_bytes)
# Derive the public key from the private key
public_key = private_key.public_key
# Get the public key bytes and skip the first byte (which is 0x04)
public_key_bytes = public_key.to_bytes()(1:)
# Compute the Keccak-256 hash of the public key bytes
keccak_hash = keccak(public_key_bytes)
# The Ethereum address is the last 20 bytes of this hash
address_bytes = keccak_hash(-20:)
# Convert the address bytes to a hexadecimal string and prepend '0x'
address="0x" + address_bytes.hex()
return address
# Example usage
private_key_hex = '0x0000000000000000000000000000000000000000000000000000000000000001'
eth_address = private_key_to_address(private_key_hex)
print("Ethereum Address:", eth_address)
Let’s convert 0x0000000000000000000000000000000000000000000000000000000000000001 to an Ethereum address. So the desired output is
0x7E5F4552091A69125d5DfCb7b8C2659029395Bdf
But increasingly
0x6579c588be2026d866231cccc364881cc1219c56
Is there something wrong with my code?