Bitcoin
bitcoinjs – Transaction transfer failed (script not found for input #0)
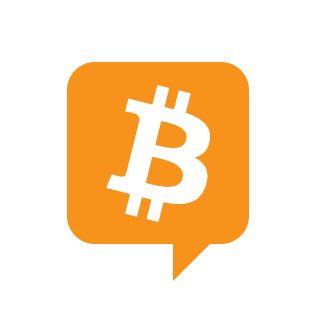
I tried making a simple BTC transfer but got the following error: Script not found for input #0. What am I missing?
const bitcoin = require("bitcoinjs-lib");
const ECPairFactory = require("ecpair");
const ecc = require("tiny-secp256k1");
const RegtestUtils = require("regtest-client");
module.exports = async function (req, res)
const regtestUtils = new RegtestUtils(
APIURL: "https://regtest.bitbank.cc/1",
APIPASS: "satoshi",
);
const network = regtestUtils.network; // regtest network params
const ECPair = ECPairFactory.ECPairFactory(ecc);
const keyPair = ECPair.fromWIF(
"xxxxxxxx"
);
const p2pkh = bitcoin.payments.p2pkh(
pubkey: keyPair.publicKey,
network,
);
const unspent = await regtestUtils.faucet(p2pkh.address, 2e4);
const txb = new bitcoin.Psbt( network );
txb.setVersion(2)
.setLocktime(0)
.addInput(
hash: unspent.txId,
index: unspent.vout,
)
.addOutput(
address: regtestUtils.RANDOM_ADDRESS,
value: 1e4,
)
.signAllInputs(keyPair);
const tx = txb.build();
console.log("hash", tx.toHex());
return;
// build and broadcast to the Bitcoin Local RegTest server
await regtestUtils.broadcast(tx.toHex());
// This verifies that the vout output of txId transaction is actually for value
// in satoshis and is locked for the address given.
// The utxo can be unconfirmed. We are just verifying it was at least placed in
// the mempool.
await regtestUtils.verify(
txId: tx.getId(),
address: regtestUtils.RANDOM_ADDRESS,
vout: 0,
value: 1e4,
);
res.status(400).json(
error: false,
message: address,
);
;