Bitcoin
How to derive a child’s private key, such as an electrum, by PHP?
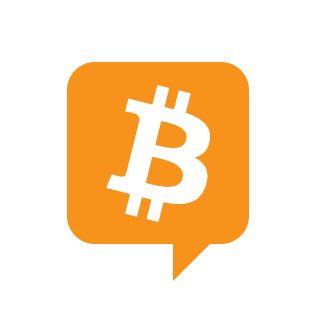
You can actually use the Bitcoin-PHP library. The following features must work.
<?php
require "vendor/autoload.php";
use BitWasp\Bitcoin\Bitcoin;
use BitWasp\Bitcoin\Key\Deterministic\HierarchicalKeyFactory;
use BitWasp\Bitcoin\Network\NetworkFactory;
use BitWasp\Bitcoin\Serializer\Key\HierarchicalKey\ExtendedKeySerializer;
use BitWasp\Bitcoin\Serializer\Key\HierarchicalKey\Base58ExtendedKeySerializer;
// Function to derive private key from a given master private key and path
function getPrivateKeyFromPath($masterKey, $path)
$network = NetworkFactory::bitcoin(); // Change to testnet if needed
// Deserialize the master key
$serializer = new ExtendedKeySerializer(new Base58ExtendedKeySerializer(Bitcoin::getMath()));
$hdFactory = new HierarchicalKeyFactory();
$key = $serializer->deserialize($network, $masterKey);
// Derive the child key for the given path
$segments = explode("https://bitcoin.stackexchange.com/", trim($path, "https://bitcoin.stackexchange.com/"));
foreach ($segments as $segment)
$index = intval($segment);
$key = $key->deriveChild($index);
return $key->getPrivateKey()->toWif($network);