Bitcoin
Mining Hardware – Why does my program crash after 30,000 iterations while performing a mining function on a blockchain?
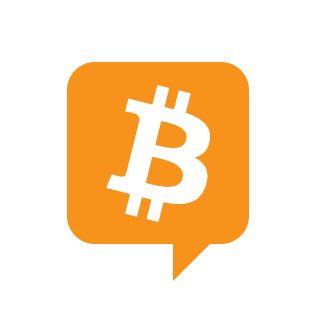
I’ve been trying to build my own blockchain from scratch in Golang.
I coded the execution layer and recently completed implementing the consensus layer for my chain.
But there is one persistent problem. There is the following function used to mine a specific block:
func (bc *Blockchain) MineBlock(b *Block) error
bc.logger.Log(
"msg", "mining block..",
)
var targetForBlock *big.Int
var err error
if (bc.Height() % HEIGHT_DIVISOR) == 0
targetForBlock, err = bc.calcTargetValue(b)
bc.Target = targetForBlock
if err != nil
return err
else
targetForBlock = bc.Target
fmt.Printf("target is %x\n", targetForBlock)
fmt.Printf("target for block %x\n", targetForBlock)
bHash := b.HashWithoutCache(BlockHasher)
hashBigInt, _ := new(big.Int).SetString(bHash.String(), 16)
for isLowerThanTarget(hashBigInt, targetForBlock) != -1
nonce := b.Header.Nonce
b.Header.Nonce++
bHash = b.HashWithoutCache(BlockHasher)
hashBigInt.SetString(bHash.String(), 16)
fmt.Printf("trying new combo with nonce %v block hash %s and target %x \n", nonce, bHash.String(), targetForBlock)
// updating timestamp
b.Header.Timestamp = uint64(time.Now().UnixNano())
b.Header.Target = targetForBlock
b.Header.NBits = targetToCompact(targetForBlock)
fmt.Printf("block mined with hash %s and target %x \n", bHash.String(), targetForBlock)
return nil
- First, the block’s target is calculated. The goal is adjusted every 5 blocks.
- Once complete, start mining. Hash the block and compare it to the target. If not, increase NONCE until the condition is resolved.
Here’s what tends to happen: After about 30,000 repetitions; My program simply crashes. I would like to know what I am doing wrong in my software architecture. The initial goals of the chain are: "0x00ffff0000000000000000000000000000000000000000000000000000"
Should I use a GPU for mining? How do I properly debug the problem?