Regenerating Bitcoin Core Signature – Bitcoin Stack Exchange
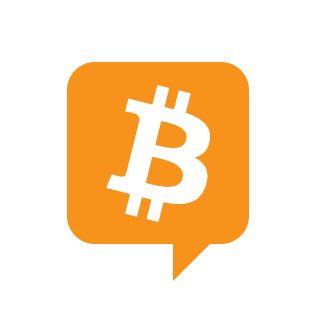
We are trying to recreate the exact same signature as Bitcoin Core. I installed bitcoin-core/secp256k1, but sometimes I get different signatures than Bitcoin Core (which is valid). I’ve read that since v0.17 there is additional entropy (32-bit little endian unsigned integer with counter). Limited experience does not allow us to fully understand and implement it.
Can someone give me some hints on how to implement this counter in my code to generate exactly the same signature as Bitcoin Core?
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <secp256k1.h>
// Function to convert hex string to byte array
void hex_to_bytes(const char *hex_str, unsigned char *byte_array, int byte_array_length)
for (int i = 0; i < byte_array_length; i++)
sscanf(hex_str + 2 * i, "%2hhx", &byte_array(i));
int main(void)
// Initialize secp256k1 context
secp256k1_context *ctx = secp256k1_context_create(SECP256K1_CONTEXT_SIGN);
// Provided private key and message hash in hex
const char *priv_key_hex = "a8188b5621448098ae66fec001acff97b7b5dcfbe371b433455135794daec37a";
const char *msg_hash_hex = "81fdf78421e2395807c9e41fa0b5ef2b587e5096d5eec43605fd669be824a872";
unsigned char priv_key(32);
unsigned char msg_hash(32);
hex_to_bytes(priv_key_hex, priv_key, sizeof(priv_key));
hex_to_bytes(msg_hash_hex, msg_hash, sizeof(msg_hash));
// Sign the transaction hash
secp256k1_ecdsa_signature signature;
secp256k1_ecdsa_sign(ctx, &signature, msg_hash, priv_key, NULL, NULL);
// Serialize the signature in DER format
unsigned char der_signature(72);
size_t der_sig_len = sizeof(der_signature);
secp256k1_ecdsa_signature_serialize_der(ctx, der_signature, &der_sig_len, &signature);
// Print the DER-encoded signature in hexadecimal
printf("DER-encoded Signature: ");
for (size_t i = 0; i < der_sig_len; i++)
printf("%02x", der_signature(i));
printf("\n");
// Free the secp256k1 context
secp256k1_context_destroy(ctx);
return 0;
data:
tx_hash: 81fdf78421e2395807c9e41fa0b5ef2b587e5096d5eec43605fd669be824a872
key1_hex: a8188b5621448098ae66fec001acff97b7b5dcfbe371b433455135794daec37a
key2_hex: 9c1fa27bdf0e6d06ab451375aa4c29ca4298af28b97ac8773200054e98a2e215
Bitcoin Core Signature: 304402203688862c2e94573a43e66305358aa74e84ace947ce080667e31265787f3738c10220380fa99f9507ae2dee832d19a7ee8fb2307708ff30e7a1532eb 30a17877fa7fe
304402204647371d0957d5456484337ff9860ab86026f05e0e97533f09126635c35a4a1302200ee59f53f4e25f2b5f2239547a8719ea58b90d98a4afcc42fe1e55 dbc70debc8
Signature with my code: 3045022100803388608a707cf1cab202a3bd758af2d42dafaf1c07ee45283b8fb478953b5002205a132280324832637d5082b8c6e42af74236647b893effe 47f72472ad277a073
3045022100a3378d3c142daead02c00d14ca69de55bcb1415e8df34173f891e7af5a7d78660220334a57a745e10d92cb007f7ddc905f3a2aa2ce72dad7b065fff339 e 4 61fa2260