Bitcoin
Verify addresses and messages using public keys, addresses, and signatures!
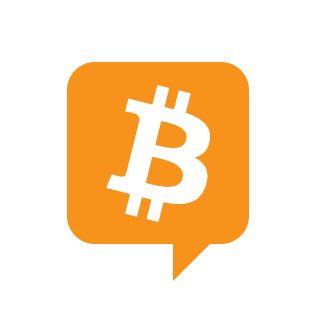
We are trying to verify ownership of a Bitcoin address using the public key, signature, and address (P2PKH, P2SH, P2WPKH, P2WSH, P2TR) as input. However, the code below does not work with Bech32 addresses.
import BitCoinLib from 'bitcoinjs-lib';
import BitCoinMessage from 'bitcoinjs-message';
BitCoinMessage.verify(message, address, signature);
As another attempt, I tried using bitcore-lib, but it doesn’t support address resolution. It accepts only the public key and signature as input.
import bitcore from 'bitcore-lib';
export function verifyMessage(publicKey, sig, text)
const message = new bitcore.Message(text);
var signature = bitcore.crypto.Signature.fromCompact(
Buffer.from(sig, "base64")
);
var hash = message.magicHash();
// recover the public key
var ecdsa = new bitcore.crypto.ECDSA();
ecdsa.hashbuf = hash;
ecdsa.sig = signature;
const pubkeyInSig = ecdsa.toPublicKey();
const pubkeyInSigString = new bitcore.PublicKey(
Object.assign(, pubkeyInSig.toObject(), compressed: true )
).toString();
if (pubkeyInSigString != publicKey)
return false;
return bitcore.crypto.ECDSA.verify(hash, signature, pubkeyInSig);
What I’m trying to achieve is similar to https://www.verifybitcoinmessage.com/, but that site also has the limitation of not being able to verify P2WPKH addresses.
Can I verify all types of addresses? If so, please provide guidance on what to do.